Introduction to Python
The AI Engineer Roadmap: Python is an incredibly powerful and easy-to-learn programming language that has taken the tech world by storm. It’s versatile, with applications in web development, data analysis, artificial intelligence, and more. Python’s syntax is clear and intuitive, making it an excellent choice for beginners looking to enter the world of programming.
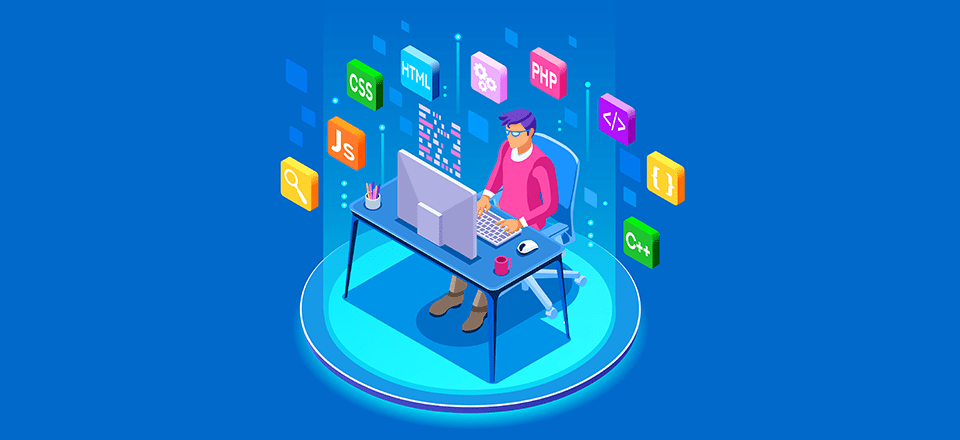
Table of Contents
Variables: Your First Step in Python Coding
Variables are essential in Python as they store data values. Think of them as labels you can attach to data, making your code more readable and maintainable. In Python, creating a variable is as simple as assigning a value to a name:
username = "Alice"
user_age = 30
Numbers: Playing with Digits
Python supports integers, floating-point numbers, and complex numbers. It provides all the standard arithmetic operations, and you can use numbers in various ways:
# Arithmetic Operations
addition = 5 + 3
division = 10 / 2
Strings: Communicate with Text
Strings are sequences of characters used to represent text. Python provides a rich set of operations and methods to manipulate strings:
greeting = "Hello, World!"
print(greeting.upper()) # HELLO, WORLD!
Lists: Keeping Your Data Organized
Lists are mutable sequences, allowing you to store an ordered collection of items. They are versatile and can be modified after creation:
colors = ["red", "green", "blue"]
colors.append("yellow")
Dictionaries: Key to Mapping Information
Dictionaries store pairs of keys and values, providing a fast way to retrieve information based on a unique key:
user_info = {"name": "Alice", "age": 30}
print(user_info["name"]) # Alice
Sets: Unique Collections
Sets are unordered collections of unique elements. They are ideal for membership testing and eliminating duplicate entries:
fruits = {"apple", "banana", "cherry"}
fruits.add("orange")
Tuples: Immutable but Essential
Tuples are immutable sequences, which means once created, their elements cannot be changed. They are perfect for storing related items:
coordinates = (10.0, 20.0)
If Condition: Making Decisions in Python
The if
statement allows you to execute code based on a condition. It’s a fundamental control structure in Python:
if user_age >= 18:
print("You are an adult.")
For Loop: Automate Repetitive Tasks
The for
loop is used to iterate over a sequence, such as a list or a string. It’s a powerful tool for automation:
for color in colors:
print(color)
Functions: Reuse Your Code
Functions are blocks of reusable code that perform a specific task. They help to make your code modular and easier to understand:
def greet(name):
return "Hello, " + name + "!"
Lambda Functions: Quick and Easy Anonymous Functions
Lambda functions are small, anonymous functions defined with the lambda
keyword. They are often used for short, simple functions:
square = lambda x: x * x
print(square(5)) # 25
Modules: Expanding Your Python Abilities
Modules are files containing Python definitions and statements. They extend the functionality of Python and can be imported into your scripts:
import math
print(math.sqrt(16)) # 4.0
Read, Write Files: Interacting with Files
Python simplifies file handling, allowing you to read from and write to files with ease. This is crucial for data storage and retrieval:
with open("example.txt", "r") as file:
content = file.read()
Exception Handling: Preparing for the Unexpected
Exception handling ensures that your program can respond to errors gracefully. The try
and except
blocks are used to catch and handle exceptions:
try:
print(10 / 0)
except ZeroDivisionError:
print("Cannot divide by zero!")
Classes and Objects: Blueprint of Python Programming
Classes provide a means of bundling data and functionality together. Creating a class defines a new type, and objects are instances of classes:
class Dog:
def __init__(self, name):
self.name = name
my_dog = Dog("Rex")
print(my_dog.name) # Rex
Conclusion: Your Python Journey Awaits
With the basics of Python under your belt, you’re ready to start exploring and creating. The journey of learning Python is ongoing, and practice is key. Dive into projects, experiment with code, and remember that every expert was once a beginner. For more information, so please visit this link.
Python Tutorials (Codebasics) on YouTube (first 16 videos) (Free)
Corey’s Python Tutorials (Free)
Codebasics python (Free)
Track B (Affordable Fees)
- Python course: for-beginner-and intermediate-learners
Assignments:
- Finish all these exercises:
- Finish exercises and quizzes for relevant topics.
- Use this LinkedIn Checklist to create a profile: Click here.
- Create a professional-looking LinkedIn profile.
Frequently Asked Questions (FAQs) : The AI Engineer Roadmap: Beginners Python Guide!
1. What is Python, and why is it popular among beginners?
- Python is a versatile and easy-to-learn programming language widely used in various fields such as web development, data analysis, artificial intelligence, etc. Its popularity among beginners stems from its clear and intuitive syntax, making it accessible for newcomers to programming.
2. How do I declare variables in Python?
- Declaring variables in Python is straightforward. You simply assign a value to a name, like this:
python username = "Alice" user_age = 30
3. What types of numbers does Python support, and how can I perform arithmetic operations?
- Python supports integers, floating-point numbers, and complex numbers. You can perform standard arithmetic operations such as addition, subtraction, multiplication, and division, like so:
python addition = 5 + 3 division = 10 / 2
4. How can I manipulate strings in Python?
- Python provides a rich set of operations and methods to manipulate strings. For example, to convert a string to uppercase, you can use the
upper()
method:python greeting = "Hello, World!" print(greeting.upper()) # Output: HELLO, WORLD!
5. What are lists, and how can I work with them in Python?
- Lists are mutable sequences that allow you to store an ordered collection of items. You can add elements to a list using the
append()
method, like this:python colors = ["red", "green", "blue"] colors.append("yellow")
6. How do dictionaries work in Python, and what are they used for?
- Dictionaries store pairs of keys and values, providing a fast way to retrieve information based on a unique key. You can access values in a dictionary by specifying the corresponding key, as shown:
python user_info = {"name": "Alice", "age": 30} print(user_info["name"]) # Output: Alice
7. What are sets, and how can they be useful in Python?
- Sets are unordered collections of unique elements, useful for tasks like membership testing and removing duplicates. You can add elements to a set using the
add()
method, like this:python fruits = {"apple", "banana", "cherry"} fruits.add("orange")
8. What are tuples, and how are they different from lists in Python?
- Tuples are immutable sequences, meaning their elements cannot be changed after creation. They are ideal for storing related items. Here’s an example of creating a tuple:
python coordinates = (10.0, 20.0)
9. How does the ‘if’ statement work in Python, and how can I use it to make decisions?
- The
if
statement allows you to execute code based on a condition. It’s fundamental for decision-making in Python, as shown in this example:python if user_age >= 18: print("You are an adult.")
10. What is a ‘for’ loop, and how can I use it to automate tasks in Python?
– The for
loop iterates over a sequence, such as a list or string, executing a block of code for each element. It’s useful for automating repetitive tasks, like so:python for color in colors: print(color)
11. What are functions in Python, and how do they help in code organization?
– Functions are blocks of reusable code that perform a specific task. They enhance code modularity and readability. Here’s how you can define and use a function:python def greet(name): return "Hello, " + name + "!"
12. What are lambda functions, and how are they different from regular functions?
– Lambda functions are small, anonymous functions defined with the lambda
keyword. They are concise and often used for simple tasks. Here’s an example of a lambda function:python square = lambda x: x * x print(square(5)) # Output: 25
13. How can I use modules to extend Python’s functionality?
– Modules are files containing Python definitions and statements. They allow you to organize code and reuse functionality across multiple scripts. You can import modules into your scripts like this:python import math print(math.sqrt(16)) # Output: 4.0
14. What are the file handling capabilities of Python?
– Python simplifies file handling, allowing you to read from and write to files effortlessly. This is essential for tasks involving data storage and retrieval. Here’s an example of reading from a file:python with open("example.txt", "r") as file: content = file.read()
15. How does exception handling work in Python?
– Exception handling ensures that your program can respond to errors gracefully. The try
and except
blocks are used to catch and handle exceptions. For instance:python try: print(10 / 0) except ZeroDivisionError: print("Cannot divide by zero!")
16. What are classes and objects in Python, and how are they used?
– Classes provide a blueprint for creating objects that bundle data and functionality together. Objects are instances of classes. Here’s an example of defining a class and creating an object:
“`python
class Dog:
def init(self, name):
self.name = name
my_dog = Dog("Rex")
print(my_dog.name) # Output: Rex
```
17. What’s the significance of practice in learning Python?
Practice is essential in mastering Python programming. By engaging in projects and experimenting with code, beginners can reinforce their understanding and gradually become proficient in Python.